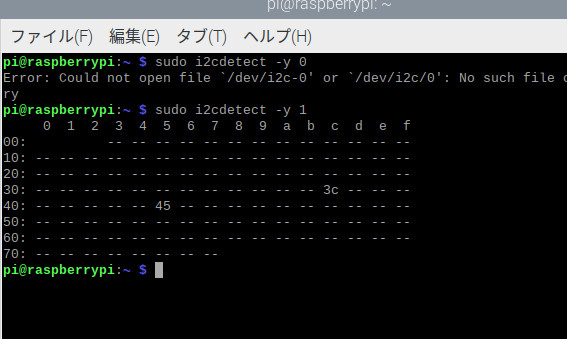
まずはSHT31からアドレスの確認
この場合は0x45がアドレス
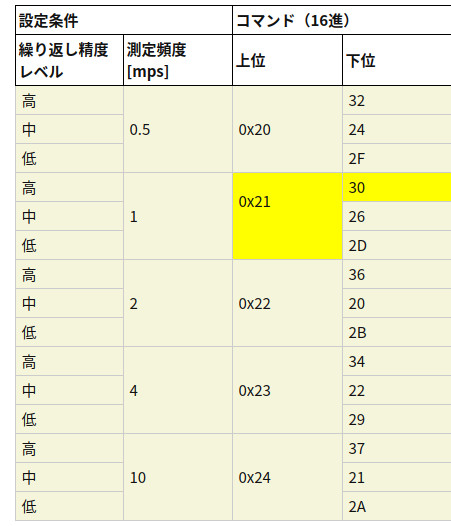
# -*- coding: utf-8 -*-
import time
import smbus
#8bitのMSBと8bitのLSBから16ビット
def mlsbChang(msb, lsb):
#msbを左に8ビットシフ下位には0がセット
#msbとlsbを論理和し16ビット化
mlsb = ((msb << 8) | lsb)
return mlsb
#データシートから
#温度
def tempChang(mlsb):
#T=-45+175*S1/(2^16-1)
t=(-45 + 175 * int(str(mlsb), 10) / (pow(2, 16) - 1))
return t
#湿度
def humidChang(mlsb):
h=(100 * int(str(mlsb), 10) / (pow(2, 16) - 1))
return h
#chanel 1
i2c = smbus.SMBus(1)
#adders 0x45
i2c_addr = 0x45
#シングルショットモード
#構成度1mps 繰りかえし高
i2c.write_byte_data(i2c_addr, 0x21, 0x30)
time.sleep(0.5)
try:
while True:
#データの取り込みコマンド 0xe0 0x00
i2c.write_byte_data(i2c_addr, 0xE0, 0x00)
#
data = i2c.read_i2c_block_data(i2c_addr, 0x00, 6)
#温度msb+温度lsb+CRC+湿度msb+湿度lsb
mlsb=mlsbChang(data[0], data[1])
t=tempChang(mlsb)
print( str('{:.4g}'.format(t)) + "C" )
mlsb=mlsbChang(data[3], data[4])
h=humidChang(mlsb)
print( str('{:.4g}'.format(h)) + "%" )
print("------")
time.sleep(1)
except KeyboardInterrupt:
pass
読み出しコマンド
i2c.write_byte_data(i2c_addr, 0xE0, 0x00)
を送るとデータがレジスタにセットされる
data = i2c.read_i2c_block_data(i2c_addr, 0x00, 6)
8ビットづつ 温度MSB 温度LSB CRC 湿度MSB 湿度LSB CRCの
順でセットされているので
温度MSBを8ビット左シフトしLSBと論理和を取って温度を取り出し
続いてADT7410のアドレスは
0x48
# -*- coding: utf-8 -*-
import smbus
import time
bus = smbus.SMBus(1)
#address 0x48
address = 0x48
register= 0x00
configration = 0x03
# 16bitに設定
bus.write_word_data(address, configration, 0x80)
#
def datachnge16(word_data):
data = (word_data & 0xff00) >> 8 | (word_data & 0xff) << 8
return data
def tempchange(data):
t=data/128.0
return t
try:
while True:
word_data = bus.read_word_data(address, register)
data = datachnge16(word_data)
t=tempchange(data)
print( str('{:.4g}'.format(t)) + "C" )
time.sleep(1)
except KeyboardInterrupt:
pass